However, the missing installation and setup instructions for development server are:
(1) download the above project sample and say put it in project folder called helloworld
(2) install google-api-python-client in the project folder helloworld
(2a) Create a file appengine_config.py in the project folder with the following contents
from google.appengine.ext import vendor
# Add any libraries installed in the "lib" folder.
vendor.add('lib')
(2b) Download google-api-python-client and related libraries into the lib sub-folder of the project folder
cd helloworld
mkdir lib
# if your Mac doesn't have pip do this -> sudo easy_install -U pip
pip install -t lib google-api-python-client
(3) Follow the instructions in here to setup OAuth 2.0 in the developer console for web app in Google App Engine Developer Console
(4) Make sure to enable Google+ API in the console or else you will get KeyError: 'id' when testing

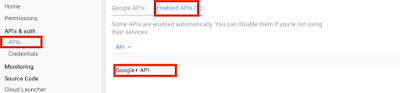
(5) Fill in Authorised Javascript origins and Authorised redirect URIs in the developer console and click create or save
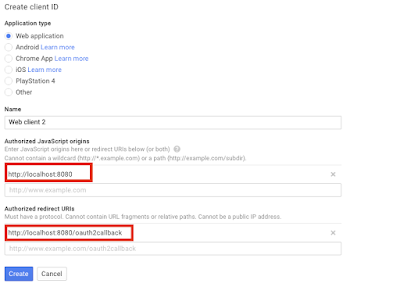
(6) copy the created Client ID and Client secret from the developer console and fill in the file client_secrets.json, like below
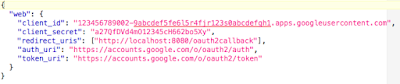
(7) Wait a few minutes and start the development server and test
Here is how to add other OAuth 2.0 apps such as Windows Live or facebook.
(1) Setup and revoke websites are :
# Google+ APIs
# Manage Application - https://console.developers.google.com
# Revoke Consent - https://www.google.com/accounts/b/0/IssuedAuthSubTokens
# OAuth 2.0 info https://developers.google.com/api-client-library/python/auth/web-app
# Windows Live API
# Manage Application - https://account.live.com/developers/applications
# Revoke Consent - https://account.live.com/consent/Manage
# OAuth 2.0 info https://msdn.microsoft.com/en-us/library/hh243647.aspx
# Facebook auth apis
# Manage Application - https://developers.facebook.com/apps/
# Revoke Consent Procedure
# Log on to Facebook.
# Click the drop-down arrow in the upper right, then select "Settings"
# Select "Apps" on the left sidebar.
# Scan the list and click the "x" on the right of any app you want to clear.
(2.1) The client_secrets_windows_live.json file is like this
{ "web": { "client_id": "0000000012345678", "client_secret": "abcdefg1lHiJK5HCnlZb-aaaaaXX", "redirect_uris": ["http://localhost:8080/windows_live_callback"], "auth_uri": "https://login.live.com/oauth20_authorize.srf", "token_uri": "https://login.live.com/oauth20_token.srf" } }
(2.2) The client_secrets_facebook.json files is like this
{ "web": { "client_id": "123456789012345", "client_secret": "a1234567e123456bc4b123456ab6543g", "redirect_uris": ["http://localhost:8080/facebook_callback"], "auth_uri": "https://www.facebook.com/dialog/oauth", "token_uri": "https://graph.facebook.com/oauth/access_token" } }
(3.1) The decorator_windows_live is like these
# Scopes are space-separated, e.g. 'wl.signin wl.basic'.
decorator_windows_live = appengine.oauth2decorator_from_clientsecrets( os.path.join(os.path.dirname(__file__), 'client_secrets_windows_live.json'), scope='wl.signin wl.basic', message=MISSING_CLIENT_SECRETS_MESSAGE)
(3.2) The decorator_facebook is like this
# Multiple Scopes should be comma-separated, e.g. 'user_about_me,email'.
decorator_facebook = appengine.oauth2decorator_from_clientsecrets( os.path.join(os.path.dirname(__file__), 'client_secrets_facebook.json'), scope='user_about_me,email', message=MISSING_CLIENT_SECRETS_MESSAGE)